如何在文本编辑器中加入c、c++文本编译成可执行程序功能呢
添加系统环境
要进行c、c++文件的编译需要用到MinGW
,查找本地路径(如: D:\Dev-Cpp\MinGW64\bin)
并将其添加到系统环境变量Path
中。
声明进程实例(MainWindow.h)
1 2 3 4
| private: bool isRunning; QProcess process;
|
运行进程(MainWindow.cpp)
构造函数中将进程相应信号与槽函数对应
1 2 3
| connect(&process,SIGNAL(finished(int)),this,SLOT(runFinished(int))); connect(&process,SIGNAL(readyReadStandardOutput()),this,SLOT(updateOutput())); connect(&process,SIGNAL(readyReadStandardError()),this,SLOT(updateError()));
|
通过环境变量中配置的编译器编译源程序文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| void MainWindow::Run(bool) { QString filePath, fileName, fileSuffix, absolutePath; if(isRunning){ process.terminate(); ui->action_Run->setIcon(QIcon(":/images/run")); return; } if(activeEditor()){ // 检查是否文件已保存 if(activeEditor()->okToContinue() && !activeEditor()->toPlainText().isEmpty()){ ui->mdiArea->activeSubWindow()->setWindowIcon(QIcon(":/images/fl")); isRunning = true; filePath = activeEditor()->getCurrentFile(); fileName = QFileInfo(filePath).baseName(); fileSuffix = QFileInfo(filePath).suffix(); absolutePath = QFileInfo(filePath).absolutePath(); buildPath= absolutePath + tr("/%1%2").arg(fileName).arg(".exe"); ui->textEdit->append(tr("Starting compile %1...").arg(filePath)); if(fileSuffix == "c"){ ui->textEdit->append(tr("gcc %1 -o %2").arg(filePath).arg(buildPath)); process.start("cmd", QStringList() << "/c" << QString(tr("gcc ")+filePath+tr(" -o ")+buildPath)); // +"& start " + buildPath }else if(fileSuffix == "cpp"){ ui->textEdit->append(tr("g++ %1 -o %2").arg(filePath).arg(buildPath)); process.start("cmd", QStringList() << "/c" << QString(tr("g++ ")+filePath+tr(" -o ")+buildPath)); } process.waitForStarted(-1); ui->action_Run->setIcon(QIcon(":/images/stop")); process.waitForFinished();//等待程序关闭 } } }
|
进程各种状态的处理槽函数
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| // 编译进程运行结束 void MainWindow::runFinished(int code){ static int run = 0; ui->action_Run->setIcon(QIcon(":/images/run")); isRunning=false; run++; // qDebug()<<code<<" "<<run<<endl; if(run%2 == 1){ ui->textEdit->append(tr("compile process exited with code %1\n").arg(code)); }else{ ui->textEdit->append(tr("run exe process exited with code %1\n").arg(code)); } // 如果返回值不为0则跳过运行程序直接加1并返回 if(code){ run++; return; }else if(!code && run%2 ==1){// 如果返回值是0(程序正常退出)且编译生成的程序还未运行过 process.start("cmd", QStringList()<<"/c"<< QString("start " + buildPath)); process.waitForStarted(-1); process.waitForFinished();//等待程序关闭 } } // 编译进程输出 void MainWindow::updateOutput(){ QString output=QString::fromLocal8Bit(process.readAllStandardOutput()); ui->textEdit->setTextColor(Qt::blue); ui->textEdit->append(output); } void MainWindow::updateError(){ QString error=QString::fromLocal8Bit(process.readAllStandardError()); ui->textEdit->setTextColor(Qt::red); ui->textEdit->append(error); process.terminate(); isRunning=false; ui->textEdit->setTextColor(Qt::blue); } // 滚动条自动往下滚动 void MainWindow::moveCurPos(){ ui->textEdit->moveCursor(QTextCursor::End); }
|
运行效果如下图:
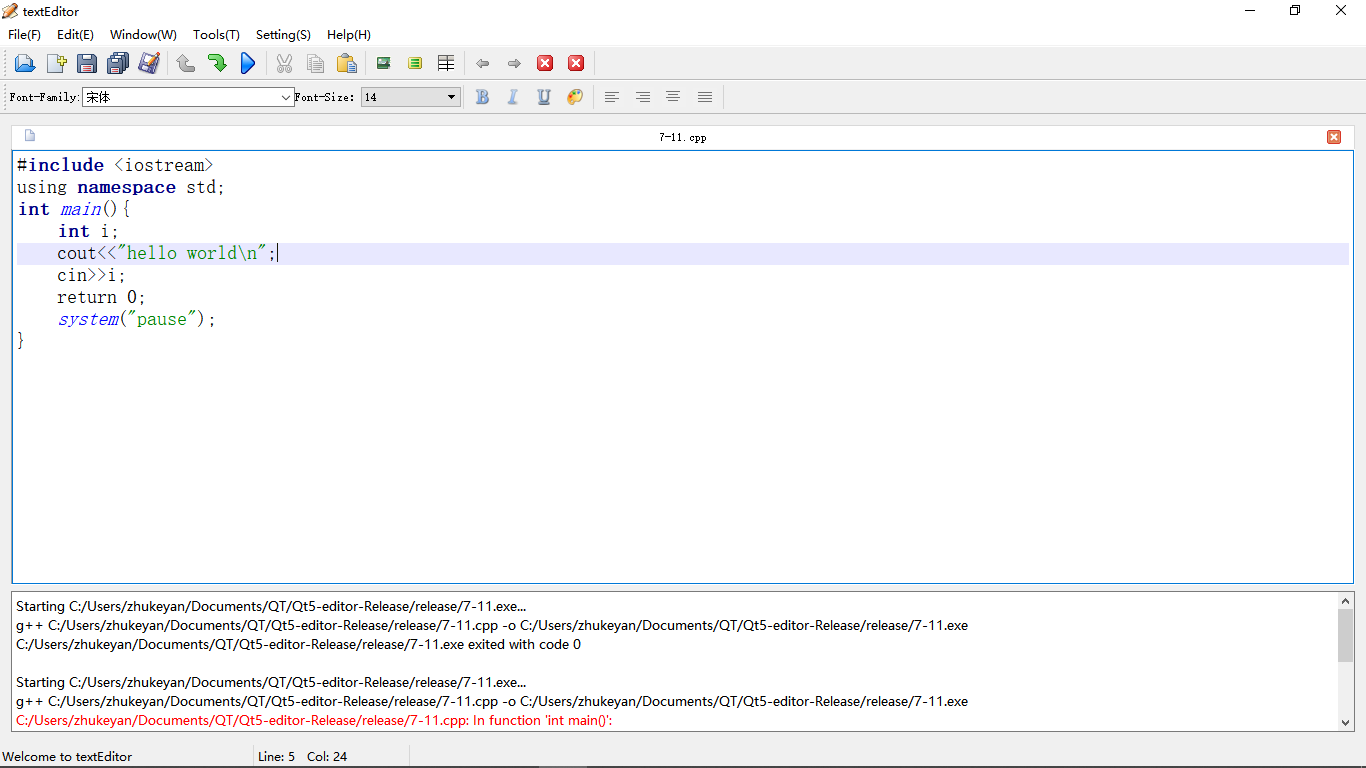
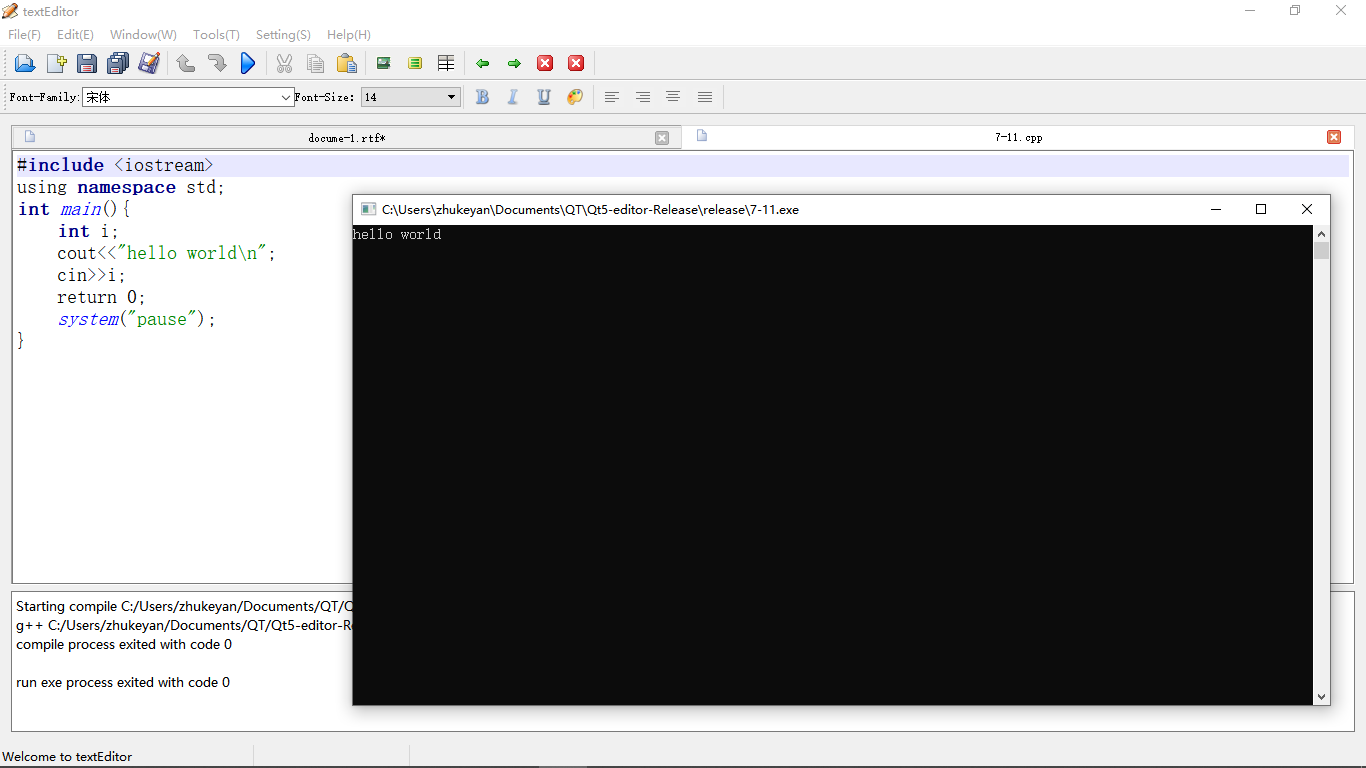